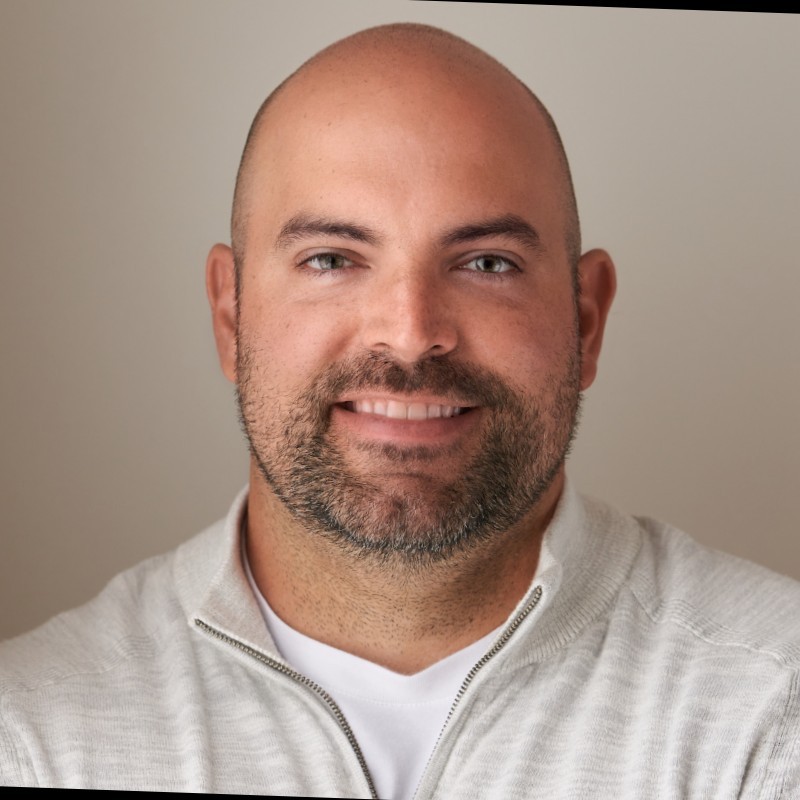
Master Stripe's "Expand" feature with this ultimate guide. Learn how to handle the "this property cannot be expanded (payment_intent)" error efficiently.
Dealing with multiple API calls to gather related data can be a real drag. If you're using Stripe, their "Expand" feature can be a game-changer. It lets you grab related object data in just one API call. This means less code, faster responses, and a smoother experience for everyone. We'll walk you through using "Expand," from the basics to handling tricky nested properties. We'll even cover how to troubleshoot those pesky errors like the dreaded "this property cannot be expanded (payment_intent)" message. Get ready to streamline your Stripe integration and simplify your data handling.
One of the most significant advantages of using Stripe's "Expand" feature is fewer API calls to retrieve related data. Without "Expand," getting details about a customer's payment method would require separate calls for each. With "Expand," you retrieve both in a single API request. This significantly speeds up data retrieval and minimizes overhead, creating a more efficient process. The Stripe API documentation notes that overusing "Expand," especially with large lists, can impact performance. Finding the right balance is key.
The "Expand" feature also simplifies data handling. Instead of navigating multiple nested objects and separate responses, you specify the exact data you need with the `expand` parameter. Need the charge details associated with a specific payment intent? A simple `expand: ['charges']` streamlines everything. This clear data handling makes your code cleaner and easier to maintain, and reduces errors. This contributes to a more efficient workflow, letting you focus on building features, not wrestling with API responses. For businesses processing high volumes of transactions, this streamlined data handling can be particularly valuable. Consider exploring automated solutions like those offered by HubiFi to further enhance your financial operations.
Faster data retrieval directly translates to a better user experience. Using the "Expand" feature lets your application load information more quickly, reducing latency for a smoother experience. No one likes waiting for a page to load, and optimizing your Stripe integration with "Expand" makes a noticeable difference. When users experience less friction, they're more likely to complete purchases and have a positive impression. "Expand" contributes to a more responsive and user-friendly application. For more details and advanced use cases, refer to the Stripe API documentation.
Stripe's "Expand" feature offers a way to retrieve related object data within a single API call, reducing the need for multiple requests. This can significantly improve the efficiency of your application, especially when dealing with complex data relationships. Think of it as a shortcut to access associated information without extra steps.
"Expand" is a query parameter you add to your Stripe API requests. It tells Stripe to include additional data about related objects directly in the response. Instead of just receiving an object ID, you get the complete object details embedded within the initial response. This is particularly helpful when you need immediate access to related data, like retrieving details about a customer associated with a payment. For more details, refer to the Stripe API reference on expanding objects.
You use the expand
parameter with an array of strings, where each string specifies the property you want to expand. For example, if you're retrieving a payment intent and want to include the payment method details, you would add expand[] = "payment_intent.payment_method"
to your request. You can expand multiple properties at once by adding more items to the expand
array, streamlining your data retrieval. Stripe allows expanding deeply nested properties using dot notation (e.g., payment_intent.payment_method
), giving you access to multi-layered relationships within your data. However, keep in mind there's a limit of four levels of nesting. One important caveat: the "expand" feature doesn't work with webhooks; you'll still need separate API calls to fetch expanded information for webhook events.
Stripe's "Expand" feature offers several advantages for developers and businesses. Let's explore some key benefits of incorporating "Expand" into your Stripe integration.
Let's move from theory to practice with a couple of real-world scenarios where using Stripe's "Expand" feature becomes essential for efficient data retrieval.
Imagine you're using the PaymentIntent.search
or PaymentIntent.list
methods to retrieve payment intents. You also need the charge details associated with each intent. Without "Expand," you'd have to make a separate API call for each intent to get its charge information. That's a lot of extra work! By including expand[] = 'data.charges'
in your initial request, you retrieve the charge details directly within the payment intent object, saving you valuable time and resources. A Stack Overflow discussion highlights this method's efficiency when working with complex data structures.
Managing subscriptions presents another common use case. When creating or retrieving a subscription, you might need access to the PaymentIntent
linked to the latest invoice. Usually, the PaymentIntent
isn't directly included in the subscription object. While you could retrieve the PaymentIntent
separately using its ID from within the LatestInvoice
object, using expand[] = 'latest_invoice.payment_intent'
is far more efficient. This way, you access the PaymentIntent
details directly within the subscription object, streamlining your workflow. This approach addresses a common challenge discussed in a GitHub issue regarding expanding PaymentIntent
when creating a subscription, showcasing "Expand's" flexibility and utility.
The expand
parameter empowers developers to retrieve related object details directly within a single API response. This eliminates the need for multiple API calls to fetch associated data, significantly reducing back-and-forth communication between your application and the Stripe API. Think of it as streamlining your grocery shopping—grabbing everything you need in one trip instead of making several separate runs. This efficiency boost saves valuable development time and minimizes the risk of exceeding Stripe's API rate limits.
"Expand" provides the flexibility to retrieve a comprehensive view of your Stripe data with minimal effort. By including multiple properties within the expand
array, you can retrieve data from various linked objects simultaneously. This means fewer API calls and a more complete picture of your transactions, customers, and other Stripe objects—all in a single request. This enhanced data retrieval simplifies data analysis and reporting, giving you a more holistic understanding of your business's financial activities.
From a developer's perspective, "Expand" simplifies working with related Stripe objects. Instead of juggling multiple API calls and managing the complexities of asynchronous operations, you can retrieve all the necessary information at once. This streamlined workflow saves time, reduces code complexity, and makes debugging and maintenance easier. However, keep in mind the nesting limit for expanded properties (currently four levels) to maintain optimal performance. The Stripe documentation offers details on expanding responses and best practices.
This section provides a practical guide to implementing Stripe's "Expand" feature, covering basic syntax, advanced techniques, and handling nested properties. By understanding these core concepts, you can optimize your Stripe integration and retrieve the data you need more efficiently.
The expand
parameter lets you include additional information in Stripe API responses. This means you can grab related data without making separate API calls, simplifying your code and reducing latency. You can expand single fields, like pulling customer details directly within a payment intent response using expand[]=customer
. This is especially helpful for quick access to related object information.
For example, imagine fetching a payment intent. Typically, you'd receive basic information about the payment. But with expand
, you can include details about the associated customer, like their email address or billing information, all in one go. This saves you from making another API call specifically for customer data. This simplifies retrieving customer information and makes your code cleaner.
When using Stripe’s API, you can specify the fields you want to expand using the expand
parameter. This parameter accepts an array of strings, where each string represents a property you want to include in the response. For instance, if you’re retrieving a payment intent and want to include the payment method details, you would add expand[] = "payment_intent.payment_method"
to your request. This streamlines data retrieval, allowing you to include multiple properties in one go and improving the efficiency of your API calls. Check out the Stripe documentation for additional information and examples.
Stripe’s API supports deeply nested expansion using dot notation, giving you access to multi-layered relationships within your data. For example, to retrieve the balance transaction associated with a charge, specify expand[] = "charges.data.balance_transaction"
. This method is incredibly useful for gathering related information without numerous API calls. However, keep in mind the nesting limit of four levels. Respecting this limit ensures efficient data handling and prevents performance issues. This feature fetches comprehensive data in a single request, simplifying data handling and improving performance, especially for complex integrations.
Expanding multiple properties at once is straightforward. Just add multiple items to the expand
array in your API request. For instance, expand[]=customer&expand[]=invoice
retrieves both customer and invoice details in a single response. This is particularly useful when you need data from several linked objects.
Let's say you're processing a refund. You might want information about the original payment, the customer who made it, and any associated invoices. With expand
, you can retrieve all of this data at once, streamlining your refund process. This approach minimizes API calls, improving your application's performance.
Stripe allows expanding deeply nested properties using dot notation, such as payment_intent.payment_method
. This lets you access properties of related objects directly. However, be mindful of the nesting limit, which is currently four levels deep. You can also expand properties within lists of objects using the data
keyword. For example, expand[]="data.payment_method"
retrieves the payment method for each item in a list of charges. This is helpful when working with lists of nested objects.
This is particularly helpful when dealing with subscriptions that involve multiple invoices. You can retrieve details about the payment method used for each invoice without making individual requests. This simplifies handling recurring billing and provides a more complete picture of the subscription's payment history. Efficient use of nested expands can significantly reduce your code's complexity when dealing with related objects in Stripe.
This section explains how to optimize your use of Stripe’s “expand” feature, ensuring your API requests are efficient and retrieve the data you need.
Before using the “expand” parameter, review Stripe’s API documentation. Knowing which fields you can expand is key. The documentation specifies which objects and properties support expansion, saving you from unnecessary trial and error. Look for the “expandable” designation next to fields. Understanding these expandable fields lets you tailor your requests to pull precisely the data you need.
When working with the Stripe API, understanding which fields you can expand is crucial for efficient data retrieval. The "expandable" label in the Stripe API documentation pinpoints these properties. This knowledge helps you tailor your requests to retrieve precisely the data you need, saving you time and reducing code complexity. It's like having a cheat sheet to navigate the Stripe API and grab exactly what you need.
Seeing this "expandable" label means you can include extra details about related objects directly in your API responses, avoiding multiple requests. For example, when retrieving a Charge object, if the customer
field is marked "expandable," you can retrieve customer details within the same API call. This eliminates a separate request to the Customer endpoint, a significant time-saver when working with numerous related objects.
To use this feature, add the expand
parameter to your API requests, specifying the fields you want to expand. You can even expand multiple fields at once for greater efficiency. For instance, when retrieving a Subscription, expand both the customer
and latest_invoice
fields to retrieve customer details and the latest invoice information simultaneously. The Stripe API reference on expanding objects offers comprehensive information and examples to guide you.
While expanding related objects in a single API call is convenient, it’s important to be mindful of performance. Expanding too many fields, especially in requests that return lists of objects, can increase response times. Stripe’s documentation often highlights performance considerations, including limits on the levels of nested expansion. Consider the trade-off between reducing the number of API calls and the potential impact on performance. If you’re dealing with large datasets, it might be more efficient to make separate, targeted requests rather than expanding everything at once. For more information on expansion best practices, check out Stripe's API reference.
One of the strengths of the “expand” feature is its ability to retrieve multiple related objects simultaneously. By using multiple expand[]
parameters in a single request, you can efficiently gather all the necessary information without making multiple API calls. For example, when retrieving customer information, you could expand both the default_source
and subscriptions
properties in one go. This streamlines your data retrieval and reduces back-and-forth communication between your application and the Stripe API. The Stripe API reference provides clear examples of how to structure these requests.
While Stripe's "Expand" feature offers significant advantages, it's essential to understand its limitations to use it effectively. Being mindful of these constraints will help you design efficient and reliable API requests.
Stripe allows you to expand nested properties using dot notation (e.g., payment_intent.payment_method
), simplifying data retrieval. However, there's a limit of four levels of nested expansion. This restriction keeps the API efficient and manageable, preventing overly complex requests that could impact performance. Keep this limit in mind when structuring your requests, especially with intricate data structures.
When using Stripe’s “Expand” feature, it’s important to be aware of the four-level nesting limit for expanded properties. This limit helps developers access related data efficiently while keeping API requests manageable and performant. Stripe uses dot notation to expand nested properties, like payment_intent.payment_method
, simplifying data retrieval. Going beyond this four-level limit, however, can create overly complex requests that slow down response times and impact your application’s overall performance.
Understanding this restriction is key to structuring efficient API requests. By staying within the four-level limit, you can ensure your application remains responsive, especially when handling complex data. Stripe’s API documentation offers further guidance on this topic and emphasizes the importance of respecting these nesting constraints for optimal API interaction.
Expanding many items, especially within a list request, can slow down response times. Retrieving related data in a single request is convenient, but overusing "Expand" can create performance bottlenecks. Carefully consider which fields you truly need and balance the benefits of fewer API calls with the potential impact on speed. Prioritize expanding only the essential data for your immediate needs. For example, if you're processing a large number of payments, you might choose to expand only the payment method details initially and fetch other related data later if needed.
When using Stripe’s “Expand” with list requests—like fetching all customers or charges—proceed with caution. Expanding many items in a list can significantly slow down response times. Imagine requesting a list of 100 customers and expanding each customer's payment method details. That's a lot of extra data being pulled in a single request. While convenient, overusing “Expand” in these scenarios can create performance bottlenecks.
Think strategically about the fields you absolutely need when expanding list requests. Balance the efficiency of fewer API calls with their potential impact on speed. If you only need a customer's email address from a large customer list, expand just that field instead of the entire customer object. For larger datasets, consider making separate, targeted requests for related data after retrieving the initial list. This keeps your list requests fast and efficient while still allowing access to related information when necessary.
The "Expand" feature doesn't work with webhooks, which provide real-time notifications about events in your Stripe account. If you need expanded information from a webhook event, you'll have to make a separate API request. This limitation requires careful planning when designing real-time data processing. Consider fetching expanded data asynchronously after receiving the webhook notification to avoid delays in your application's response. For instance, if you need customer details when a new subscription is created, you can use the customer ID provided in the webhook event to fetch the expanded customer data in a separate request.
Occasionally, you might encounter the frustrating "this property cannot be expanded (payment_intent)" error. This typically occurs when you're trying to retrieve related data, like fees or charges, directly within a Payment Intent object, but the API doesn't support direct expansion for that specific property. Let's break down why this happens and how to work around it.
Not all properties within Stripe objects are expandable. The expand
parameter works well in many scenarios, but certain properties, particularly within nested objects or when using specific API calls like PaymentIntent.search
or PaymentIntent.list
, may not support direct expansion. This limitation often stems from how the API is structured and optimized for performance. For instance, expanding deeply nested objects for every item in a large list could significantly impact response times. Discussions on Stack Overflow highlight similar challenges developers face when trying to retrieve additional details, like fees, from PaymentIntents within search results.
Sometimes, discrepancies between the Stripe documentation and the actual API behavior can contribute to this issue. For example, the Stripe .NET library has encountered situations where the PaymentIntent
object isn't directly expandable when creating a subscription, even though the documentation might suggest otherwise. These inconsistencies can be due to various factors, including API versioning or specific library implementations. For businesses dealing with high-volume transactions, these nuances can become particularly important for maintaining efficient data retrieval.
Don't worry, encountering a non-expandable property isn't a dead end. There are practical workarounds to retrieve the information you need. While it might require an extra step or two, these methods ensure you can still access the necessary data. For companies prioritizing streamlined financial operations, understanding these workarounds is crucial.
If direct expansion isn't an option, the most common workaround is to retrieve the related object separately using its ID. Often, the parent object will contain the ID of the related object you're interested in. For example, if you can't directly expand the PaymentIntent
from a subscription object, you can likely find the PaymentIntent
ID within the subscription or related invoice data. You can then use this ID to make a separate API call to retrieve the full PaymentIntent
object and its associated details. While this approach might seem less efficient than direct expansion, it's a reliable way to access the information you need. Developers on GitHub have shared similar experiences and confirmed this method as a practical solution. This method is particularly relevant for businesses that need to reconcile large volumes of transactions quickly and accurately.
Let's say you need to retrieve the charges associated with a Payment Intent. If direct expansion of the charges
property isn't working, you can retrieve the PaymentIntent
object first. Once you have the PaymentIntent
, you can then use its ID to make a separate request to list the charges associated with that specific PaymentIntent
. This two-step process ensures you get the required information, even if direct expansion isn't supported. This Stack Overflow thread provides a helpful example of how to retrieve expanded data, like charges, from PaymentIntents using the expand: ['data.charges']
parameter with the PaymentIntent.list
or PaymentIntent.search
methods. This level of detailed transaction data is essential for businesses looking to gain deeper insights into their revenue streams and customer behavior. For companies seeking automated solutions for revenue recognition, HubiFi offers tools that can seamlessly integrate with your Stripe data to provide real-time analytics and ensure compliance.
High-volume businesses face unique challenges. Processing tons of transactions requires a robust and efficient system. Let's explore some best practices to ensure your business can handle the load.
Think big from the start. Anticipate future growth in your user base and data volume during your initial design phase. This forward-thinking approach will save you headaches down the road. A modular architecture is key. By structuring your application into independent modules, you can scale specific components as needed without impacting the entire system. This flexibility is crucial for adapting to changing demands. Consider features like horizontal scaling, where you add more servers to distribute the load, ensuring your system remains responsive even during peak times. For growing businesses, choosing the right architecture is a foundational step towards long-term success.
Quick and efficient data retrieval is essential for high-volume businesses. Load balancing distributes incoming requests across multiple servers, preventing overload and ensuring consistent performance. Think of it as a traffic controller for your data, directing requests to the least busy server. Caching frequently accessed data is another powerful technique. By storing this data in a readily accessible location, you can significantly reduce database load and improve response times, leading to a smoother user experience. Explore our integrations at HubiFi to see how we connect with various platforms for seamless data flow.
Your database is the heart of your operations, so optimizing its performance is critical. Load balancing plays a vital role here, distributing the workload across multiple database servers. Caching is equally important, especially for frequently accessed data. Consider implementing database indexing to speed up data retrieval. Regularly analyze your database queries to identify and optimize any bottlenecks. For high-volume businesses, these optimizations can significantly impact efficiency and customer satisfaction. For more insights on financial operations, visit the HubiFi blog and learn how we help businesses like yours. You can also explore our pricing information to see how our solutions fit your budget.
Occasionally, you might hit a few snags with Stripe’s “Expand” feature. This section covers common errors, debugging strategies, and how to implement robust error handling to keep your integration running smoothly.
One frequent issue is trying to expand properties that don’t exist or aren’t expandable for a specific object. Always double-check the Stripe API documentation to confirm which properties you can expand for the object you’re querying. For example, you can't expand a customer's favorite_color
if that property isn't defined. Referencing the documentation will save you time and frustration. If you’re seeing unexpected results, make sure your API version is up-to-date, as expandable properties can change between versions.
If your “Expand” requests aren’t working correctly, start by checking the API response for error messages. These messages often pinpoint the problem. For instance, if you’re working with nested properties, ensure you’re using the correct dot-notation in your expand
parameter, like expand: ["setup_intent.payment_method"]
. This Stack Overflow discussion provides a helpful example. Also, verify that you’re using the correct object IDs in your API calls – a simple typo can throw things off. Using a debugging tool or logging your requests and responses can provide valuable insights.
Solid error handling is essential when using “Expand.” Wrap your API calls in try-catch
blocks to handle potential issues gracefully. The Stripe API generally provides descriptive error messages, so log these for analysis and debugging. Consider fallback mechanisms or user notifications if an expansion request fails. For example, if you can’t expand a customer’s billing details, you might display a generic message and let the user manually enter the information. This prevents a frustrating user experience. Testing different error scenarios will help you build a more resilient integration.
After integrating Stripe's Expand feature, the next step is ensuring its ongoing success and adaptability. This involves tracking key metrics, staying informed about API updates, and anticipating future enhancements. A well-structured approach to these areas can significantly contribute to the long-term effectiveness of your integration.
Clear performance metrics are essential for gauging the success of your Stripe Expand integration. Think about what you want to achieve with this integration. Is it faster processing times? Reduced API calls? Pinpointing these goals upfront allows you to select the most relevant metrics. For example, you might track the reduction in API call volume, the improvement in page load speeds, or the decrease in database query times. Using analytics solutions, like those offered by HubiFi, can provide valuable insights into these areas, giving you a clear picture of your integration's impact. Consistently monitoring these metrics helps identify areas for optimization and ensures your integration continues to deliver value.
APIs evolve, and Stripe is no exception. Staying informed about API changes is crucial for maintaining a functional and compliant integration. Regularly review Stripe’s API documentation and subscribe to their developer updates. This proactive approach will help you anticipate potential disruptions and adapt your integration accordingly. Think of it as routine maintenance for your code—a small investment of time that can prevent major headaches down the road.
Looking ahead is key to future-proofing your integration. Stripe regularly introduces new features and enhancements. By anticipating these changes, you can prepare your systems and processes to take full advantage of them. A good starting point is to follow industry trends and engage with the Stripe developer community. Establishing a standard training process for your team can also be beneficial. This ensures everyone stays informed about new features and best practices, reducing the risk of non-compliance and maximizing the benefits of future Stripe updates. This proactive approach will not only keep your integration running smoothly but also position your business to leverage the latest advancements in payment processing. For more insights on optimizing financial operations, explore the HubiFi blog.
Stripe's "Expand" feature offers more than just streamlined API requests; it unlocks practical applications that can significantly impact your business operations, particularly for high-volume companies. Let's explore how "Expand" can revolutionize financial operations, compliance processes, and customer data management.
For businesses processing a high volume of transactions, "Expand" can be instrumental in automating key financial processes. By retrieving related data in a single API call, you can automate reconciliation, reporting, and revenue recognition. This reduces manual data gathering and improves the accuracy of your financial data. Automating these routine compliance tasks with solutions like those offered by HubiFi can significantly lower your operational costs, freeing up your team to focus on strategic initiatives. As highlighted in this article on financial performance metrics, focusing on key metrics like revenue growth and cost management is crucial for driving efficiency and profitability. "Expand" empowers you to access these metrics more efficiently.
Maintaining compliance with industry regulations, such as ASC 606 and ASC 944, can be complex. "Expand" simplifies this by enabling you to retrieve all necessary data for compliance reporting in a single request. This streamlines your compliance processes and reduces the risk of errors or omissions. By integrating compliance requirements directly into your operations, you can enhance efficiency and improve overall performance. Standardizing your training and compliance processes, becomes easier with the comprehensive data access provided by "Expand." This ensures your team stays informed about regulatory changes and minimizes the risk of violations.
Effective customer data management is essential for personalized experiences and targeted marketing. "Expand" allows you to retrieve comprehensive customer data, including transaction history, subscription details, and associated metadata, with a single API call. This eliminates the need for multiple requests, improving the efficiency of your customer relationship management (CRM) system. Seamless communication between systems is key to reducing data silos and improving data accessibility, which enables better analytical results and more strategic planning. Furthermore, building scalable applications that can handle increasing data volume is crucial for long-term success. "Expand" contributes to scalability by optimizing resource usage and ensuring your application can handle growth efficiently.
What exactly is expanding a Stripe object?
Expanding a Stripe object is like asking for extra details all at once. Instead of just getting a simple ID for a related object (like a customer linked to a payment), you get all the important information about that customer right then and there, within the same API response. It's a way to avoid making multiple back-and-forth trips to the Stripe server.
Why should I use the "Expand" feature?
It boils down to efficiency and cleaner code. "Expand" reduces the number of API calls you need to make, which speeds up your application and reduces the chances of hitting Stripe's rate limits. It also simplifies your code by fetching related data upfront, so you don't have to manage multiple requests and responses.
Are there any downsides to using "Expand"?
While generally beneficial, overuse of "Expand" can sometimes slow down response times, especially if you're expanding many fields or dealing with large lists. Also, be aware of the nesting limit (four levels deep) for expanded properties. Finally, remember that "Expand" doesn't work with webhooks.
How can I troubleshoot "Expand" if I encounter problems?
First, double-check the Stripe API documentation to ensure you're trying to expand valid properties. Look for error messages in the API responses, which often provide clues about the issue. Logging your requests and responses can also help pinpoint the source of the problem.
Where can I find more information about best practices for "Expand," especially for high-volume businesses?
Stripe's API reference is your go-to resource for detailed information and examples. For high-volume businesses, consider focusing on efficient data retrieval strategies, database optimization, and staying up-to-date with API changes to ensure your integration remains performant and scalable. HubiFi's blog and resources also offer valuable insights into optimizing financial operations and data management for growing businesses.
Former Root, EVP of Finance/Data at multiple FinTech startups
Jason Kyle Berwanger: An accomplished two-time entrepreneur, polyglot in finance, data & tech with 15 years of expertise. Builder, practitioner, leader—pioneering multiple ERP implementations and data solutions. Catalyst behind a 6% gross margin improvement with a sub-90-day IPO at Root insurance, powered by his vision & platform. Having held virtually every role from accountant to finance systems to finance exec, he brings a rare and noteworthy perspective in rethinking the finance tooling landscape.